Mastering Console Methods in JavaScript

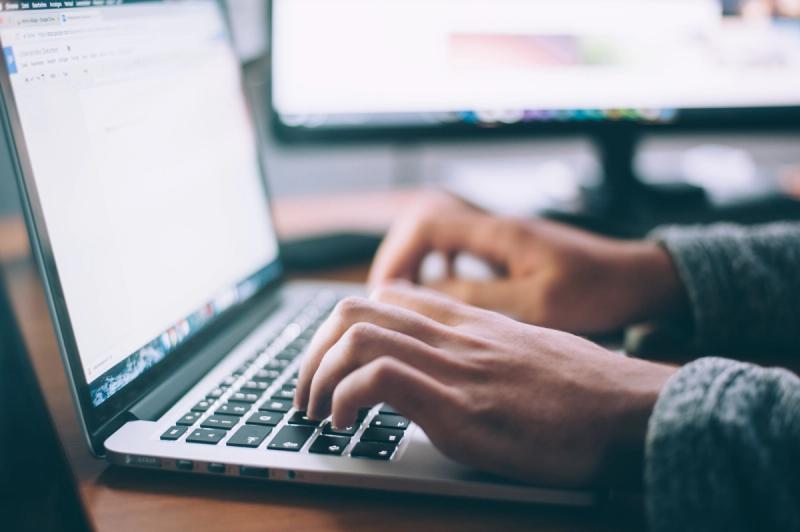
Welcome to my website! Are you looking to improve your JavaScript coding skills and streamline your development process? If so, here's a great post to start mastering the console methods. The console object is a powerful tool that allows developers to print messages, debug code, and optimize performance. In this blog, we'll explore the basics and advanced features of console methods, and show you how to use them effectively for debugging and performance optimization. let's get started!
JavaScript is a powerful language that can be used to create dynamic and interactive web applications. One of the key features of JavaScript is the ability to output messages to the console, which can be extremely helpful for debugging and performance optimization. The console object in JavaScript provides developers with several methods to log messages to the console, including log()
, error()
, alert()
, info()
, clear()
, and many more.
The console object is an important tool for developers because it provides a way to output messages and data to the browser console, which is a built-in tool in most modern web browsers. It allows developers to see real-time feedback from their code and helps them quickly identify and fix errors. The console can also be used to measure the performance of code and optimize it for faster execution.
In this article, we'll explore the various console methods available in JavaScript and provide examples of how they can be used for debugging and performance optimization. By the end of this article, you will have a complete understanding of the Console object in JavaScript and how to use it effectively in your web development projects.
Basic Console Methods
Native console methods are one of the most used and important tools for developers in JavaScript. They allow you to quickly and easily output information to the console, which can be extremely useful for debugging and troubleshooting your code.
Here are the five basic console methods:
console.log()
The console.log()
method is used to log messages to the console. It is an essential tool for debugging and troubleshooting, as it allows you to have information about the state of your code at various points during execution.
Here's an example of using console.log()
to output a message to the console:
console.log("Hello, world!");
console.error()
The console.error()
method is used to output error messages to the console. It's similar to console.log()
, but it's usually used to log more serious errors that may cause your code to fail.
Here's an example of using console.error()
to output an error message to the console:
console.error("Oops, something went wrong!");
console.warn()
The console.warn()
method is used to output warning messages to the console. It's similar to console.log()
and console.error()
, but it's usually used to log warnings about potential issues in your code.
Here's an example of using console.warn()
to output a warning message to the console:
console.warn("Warning: This function is deprecated!");
console.info()
The console.info()
method is used to output informational messages to the console. It's similar to console.log()
, but it's usually used to log messages that provide additional information about your code.
Here's an example of using console.info()
to output an informational message to the console:
console.info("This is a message with additional information.");
console.clear()
The console.clear()
method is used to clear the console. It's a simple tool that can be useful for keeping your console clean and organized during development.
Here's an example of using console.clear()
to clear the console:
console.clear();
Advanced Console Methods
While basic console methods like console.log()
and console.error()
are useful for outputting messages to the console, there are several more advanced console methods that can help you with debugging and performance profiling. Here are some of the most useful advanced console methods:
console.assert()
The console.assert()
method checks if an assertion is true and outputs an error message to the console if it's not. The method takes two arguments: the first argument is the assertion to test, and the second argument is an optional error message to display if the assertion fails.
Here's an example usage of console.assert()
:
let num = 10;
console.assert(num > 20, "num is not greater than 20");
In this example, the assertion will fail since num
is not greater than 20, and the message "num is not greater than 20" will be logged to the console.
console.table()
The console.table()
method takes an array or an object as its argument and logs it to the console as a table. This method is useful for visualizing complex data structures and quickly identifying patterns or anomalies.
Here's an example usage of console.table()
:
const users = [
{ name: "Alice", age: 25 },
{ name: "Bob", age: 30 },
{ name: "Charlie", age: 35 }
];
console.table(users);
In this example, the users
array will be logged to the console as a table with columns for "name" and "age".
console.time() and console.timeEnd()
The console.time()
and console.timeEnd()
methods are used to measure the time it takes for a section of code to run.
Here's an example:
console.time('myFunction');
// some code to measure
console.timeEnd('myFunction');
In this example, the console.time()
method starts a timer with the label "myFunction", and the console.timeEnd()
method stops the timer and outputs the time it took for the code in between to run.
console.group() and console.groupEnd()
When you have a series of related console messages that you want to group together, you can use the console.group()
and console.groupEnd()
methods to create a collapsible group in the console.
Here's an example:
console.group('User Info');
console.log('Name: John Doe');
console.log('Email: john.doe@example.com');
console.groupEnd();
In this example, the console.group()
method starts a new console group with the label "User Info", and the console.groupEnd()
method ends the group. Any console messages logged between the two methods will be indented and displayed under the group label.
console.trace()
The console.trace()
method outputs a stack trace to the console, showing the function calls and their locations that led up to the current line of code. This method is useful for debugging complex code with multiple function calls.
Here's an example:
function myFunction() {
console.trace();
}
function anotherFunction() {
myFunction();
}
anotherFunction();
In this example, the console.trace()
method is called from the myFunction()
function, which is called from the anotherFunction()
function, which is called from the global scope. The console outputs a stack trace showing the function calls and their locations in the code.
Tips and Tricks for Using Console Methods
Console methods can be used in many different ways to help developers debug and optimize their code. Here are some tips and tricks for getting the most out of console methods in JavaScript:
Using string substitutions to format console output
String substitutions allow you to dynamically insert values into a console message. To use string substitutions, include %s
in the message string where you want to insert a value, and then pass the value as an argument to the console method.
For example:
const name = "John";
const age = 30;
console.log("My name is %s and I am %d years old", name, age);
This will output the message "My name is John and I am 30 years old
" to the console.
Using the console object in different contexts (e.g. in the browser console or in Node.js)
The console object is available in different contexts, such as the browser console or in Node.js. In the browser console, you can use console methods to interact with the webpage and debug client-side JavaScript code. In Node.js, you can use console methods to debug server-side JavaScript code. It's important to be familiar with the different contexts in which you might use the console object and how to use console methods in those contexts.
Using console methods to debug code and catch errors
Console methods are a powerful tool for debugging code and catching errors. You can use console.log() to output the value of a variable or the result of a function call to the console to help you understand what's happening in your code. You can also use console.assert()
to check if an assertion is true and output an error message if it's not. This can help you catch errors early in the development process.
Best practices for using console methods in production code
While console methods are a useful tool for debugging and development, it's important to use them judiciously in production code. Including too many console messages in production code can affect performance and can expose sensitive information. To use console methods effectively in production code, consider using a logging library that allows you to control the verbosity and severity of log messages. Also, make sure to remove console messages from production code before deploying it to production.
Conclusion
In conclusion, mastering the console methods in JavaScript is an important skill for any developer who wants to write efficient and effective code. In this article, we've covered both basic and advanced console methods, including console.log()
, console.table()
, and console.assert()
, along with some tips and tricks for using these methods effectively. Tricks are also included. Tricks are also included.
By mastering console methods, you'll be able to easily debug your code and catch errors, measure your application's performance, and output useful information to the console for your own or other developers' use.
In addition, using console methods can help you write more readable and maintainable code, providing a way to organize and group related console messages, as well as to optimize output using string replacement. customizes. Format.
In short, console methods are a powerful tool in any JavaScript developer's arsenal, and by taking the time to master them, you'll be able to write better code, debug more efficiently, and ultimately be a more effective developer.
Table of Contents
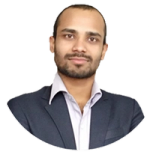
Related Posts

