How to Create a Splash Screen in Next.js

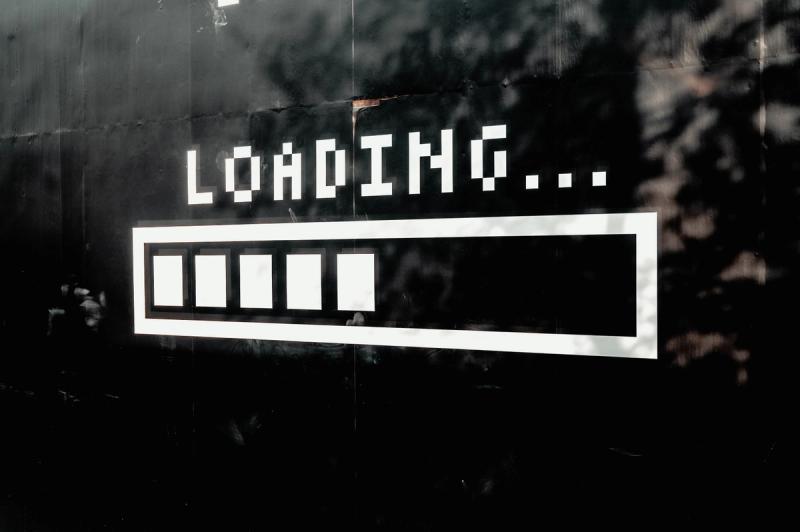
Hello and welcome to my website!
If you're a web developer working with Next.js, you know that creating a seamless user experience is key. One way to achieve this is by adding a loading screen to your application. A loading screen can help keep your users engaged and informed while your application loads data and assets in the background.
In this tutorial, we'll walk you through how to add a loading screen to your Next.js application. Whether you're building a single-page application or a multi-page website, we'll provide step-by-step instructions to help you implement a loading screen that works for your specific use case.
- To get more tips like this about Next.js and React follow me here
What is Next.js
Next.js is a popular open-source framework for building server-side rendered React applications. It was created by Vercel and is widely used by developers to build fast and scalable web applications.
Next.js provides a variety of features that make it easy to create high-performance web applications, including server-side rendering, automatic code splitting, static site generation, and more. Additionally, it has a robust ecosystem of plugins and libraries that can help you extend its functionality and customize your application to your specific needs.
Overall, Next.js is a powerful tool for building modern web applications that can help you save time and streamline your development process. Whether you're a seasoned developer or just getting started with web development, Next.js is definitely worth checking out.
Let’s go to the topic 🚀
First we are going to create or update _app.jsx in /pages that will look like this.
You can paste the following content:
import React, { useState, useEffect } from "react";
import { useRouter } from "next/router";
import "../styles/globals.css";
import Script from "next/script";
import Head from "next/head";
export default function App({ Component, pageProps }) {
const router = useRouter();
const [pageLoading, setPageLoading] = useState(false);
useEffect(() => {
const handleStart = () => {
setPageLoading(true);
};
const handleComplete = () => {
setPageLoading(false);
};
router.events.on("routeChangeStart", handleStart);
router.events.on("routeChangeComplete", handleComplete);
router.events.on("routeChangeError", handleComplete);
}, [router]);
return (
<>
{pageLoading ? (
<div>
<section class="dots-container">
<div class="dot"></div>
<div class="dot"></div>
<div class="dot"></div>
<div class="dot"></div>
<div class="dot"></div>
</section>
</div>
) : (
<Component {...pageProps} />
)}
</>
);
}
Secondly we are going to create loader.css in /styles that will content a CSS of our loader.
.dots-container {
display: flex;
align-items: center;
justify-content: center;
height: 100%;
width: 100%;
}
.dot {
height: 20px;
width: 20px;
margin-right: 10px;
border-radius: 10px;
background-color: #b3d4fc;
animation: pulse 1.5s infinite ease-in-out;
}
.dot:last-child {
margin-right: 0;
}
.dot:nth-child(1) {
animation-delay: -0.3s;
}
.dot:nth-child(2) {
animation-delay: -0.1s;
}
.dot:nth-child(3) {
animation-delay: 0.1s;
}
@keyframes pulse {
0% {
transform: scale(0.8);
background-color: #b3d4fc;
box-shadow: 0 0 0 0 rgba(178, 212, 252, 0.7);
}
50% {
transform: scale(1.2);
background-color: #6793fb;
box-shadow: 0 0 0 10px rgba(178, 212, 252, 0);
}
100% {
transform: scale(0.8);
background-color: #b3d4fc;
box-shadow: 0 0 0 0 rgba(178, 212, 252, 0.7);
}
}
Thirdly we need to inject this CSS code into the header of our application so that it is loaded by the browser as quickly as possible.
To do this update or create _app.js of your application to look like this:
import React, { useState, useEffect } from "react";
import { useRouter } from "next/router";
import "../styles/globals.css";
import "../styles/loader.css";
import Script from "next/script";
import Head from "next/head";
export default function App({ Component, pageProps }) {
const router = useRouter();
const [pageLoading, setPageLoading] = useState(false);
useEffect(() => {
const handleStart = () => {
setPageLoading(true);
};
const handleComplete = () => {
setPageLoading(false);
};
router.events.on("routeChangeStart", handleStart);
router.events.on("routeChangeComplete", handleComplete);
router.events.on("routeChangeError", handleComplete);
}, [router]);
return (
<>
{pageLoading ? (
<div>
<section class="dots-container">
<div class="dot"></div>
<div class="dot"></div>
<div class="dot"></div>
<div class="dot"></div>
<div class="dot"></div>
</section>
</div>
) : (
<Component {...pageProps} />
)}
</>
);
}
If you are in local, you can limit internet connection of you browser in console to 2G to see the loader below:
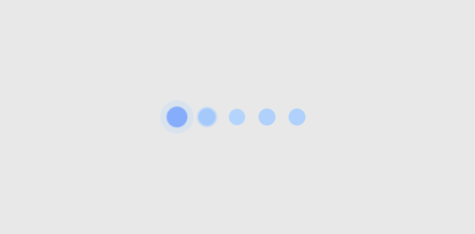
Conclusion
In conclusion, adding a loading screen to a Next.js application is a simple and effective way to enhance the user experience. By following the steps outlined in this guide, you can easily create a loading screen that will display while your application is loading. Utilizing a loading screen can not only improve the visual appeal of your application but also reduce frustration for users who may experience slower loading times. With Next.js, adding a loading screen is a straightforward process that can make a big difference in the overall user experience of your application.
Table of Contents
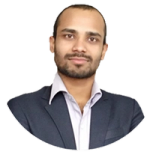